How to Create a Blog with Astro
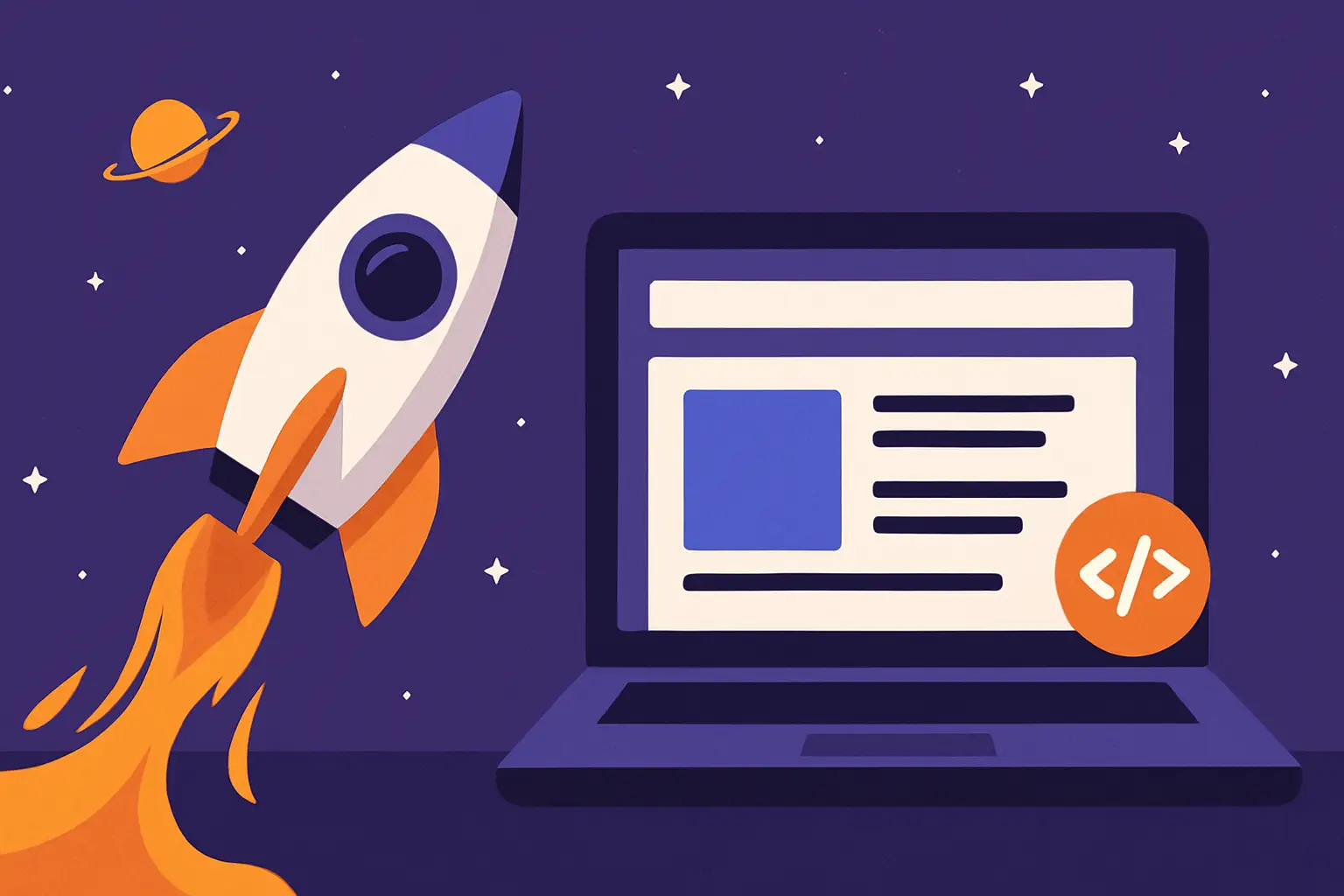
Astro is a modern static site generator that makes building fast and optimized websites easy. In this guide, we’ll walk through the steps to create a blog using Astro.
Prerequisites
- Node.js installed on your machine
- Basic knowledge of JavaScript and web development
Step 1: Create a New Astro Project
Run the following command to create a new Astro project:
npm create astro@latest
Follow the prompts to set up your project. Choose a blog template if available, or start with a basic template.
Step 2: Install Dependencies
Navigate to your project directory and install the dependencies:
cd your-project-name
npm install
Step 3: Set Up Blog Content
Create a src/content
directory to store your blog posts. Use Markdown files (.md
) for each post. For example:
src/content/posts/my-first-post.md
Example Markdown File
---
title: "My First Blog Post"
date: "2023-10-01"
description: "An introduction to my new blog."
---
Welcome to my blog! This is my first post using Astro.
Step 4: Create a Blog Layout
In the src/layouts
directory, create a layout file for your blog posts, e.g., BlogLayout.astro
:
---
const { children, frontmatter } = Astro.props
---
<article>
<h1>{frontmatter.title}</h1>
<p>{frontmatter.date}</p>
<div>{children}</div>
</article>
Step 5: Display Blog Posts
Create a page to list all blog posts. For example, in src/pages/blog.astro
:
---
import { getCollection } from 'astro:content';
const posts = await getCollection('posts');
---
<h1>Blog</h1>
<ul>
{posts.map(post => (
<li>
<a href={`/posts/${post.slug}`}>{post.data.title}</a>
</li>
))}
</ul>
Step 6: Run Your Blog Locally
Start the development server:
npm run dev
Visit http://localhost:3000
to see your blog in action.
Step 7: Deploy Your Blog
Astro supports deployment to platforms like Vercel, Netlify, and GitHub Pages. Build your site with:
npm run build
Then follow the deployment instructions for your chosen platform.
Conclusion
You’ve successfully created a blog using Astro! Customize it further by adding styles, components, and features to make it your own.